Decouple Data from UI with React Hooks
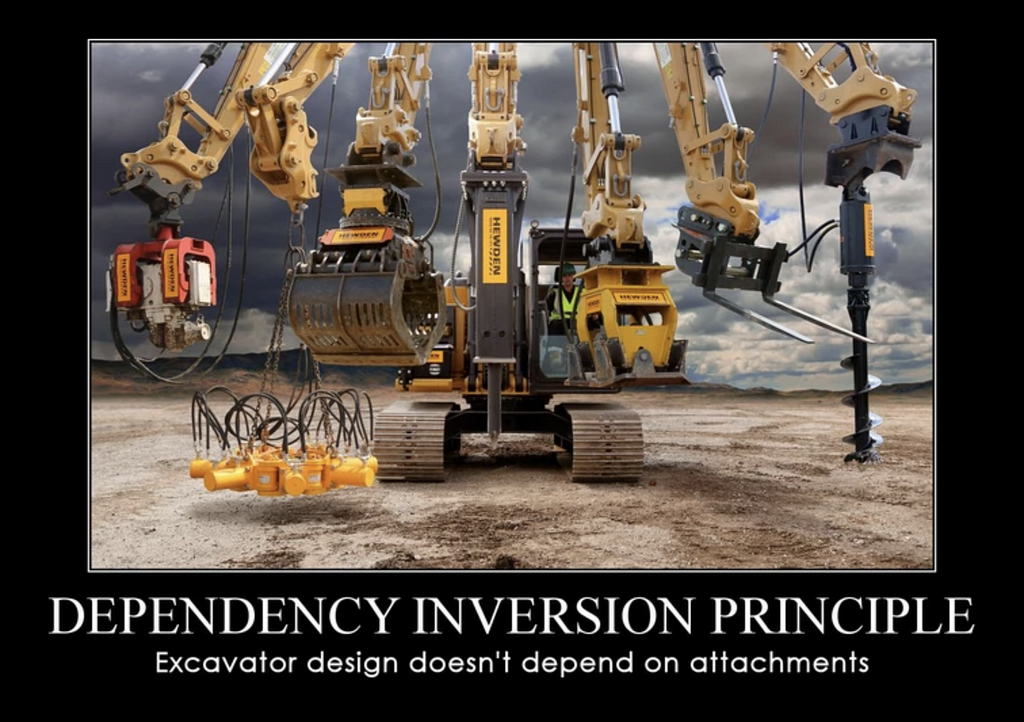
I‘m certain you have seen (or written) this common React pattern: (a) render a placeholder/ loader/spinner while some data is fetched via AJAX, then (b) re-render the component based on the data received. Let’s write a functional component leveraging the Fetch API to accomplish this.
Let’s say my app grows, and there are X components that use the same data fetching logic because… reasons. To avoid spamming the server with data requests, I decide to use Local Storage to cache the data.
OK… does that mean I need to update the data logic X times? 😬😱
Nope, let’s DRY it up by writing a custom hook useSomeData
.
The components that share this data logic now look concise.
OK… DRY code is great, but so what?
Let’s say my app becomes complex, so I decide to use Redux to handle AJAX requests and maintain a global app state. I simply update the implementation of useSomeData
without touching the UI components.
Then GraphQL comes along and I jump on the bandwagon. Again, I simply update the implementation of useSomeData
without touching the UI components.
Rinse and repeat whenever I’m compelled to update the data layer with the latest/hottest state management framework or API paradigm.
To me, this looks a lot like the classic Dependency Inversion Principle, the “D” in SOLID (check out this excellent explainer by Matthew Lucas). While this is not OOP by any means, where we formally define an abstract Interface
and create a concrete Class
that implements that Interface
, I would argue that there is a de facto “interface” that useSomeData
provides to the various UI components using it. In this example, the UI doesn’t care how useSomeData
works, as long as it receives someData
, loading
, and error
from the hook.
So in theory, this frees the UI from being locked into any particular implementation of the data layer, and enables migrating to new implementations (frameworks/libraries/etc) without having to update the UI code, as long as the “interface” contract is honored.
Curious to hear your thoughts.
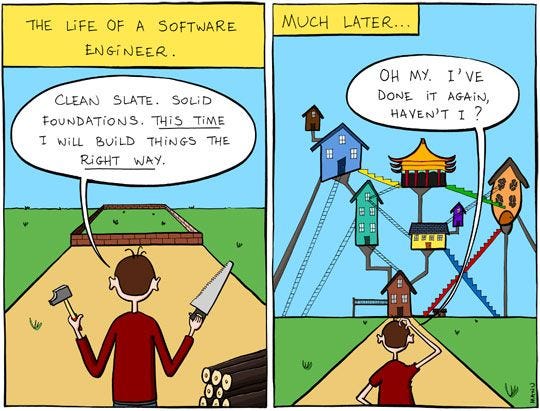
P.S. The Container pattern, Render Props, and HOC are popular options to decouple the data layer from the UI layer for classical components. This article is not meant to be a debate as to whether Hooks is better or worse. I’m simply sharing how I learned to use Hooks to apply the same separation of concerns.